Laravel - Ressource CRUD
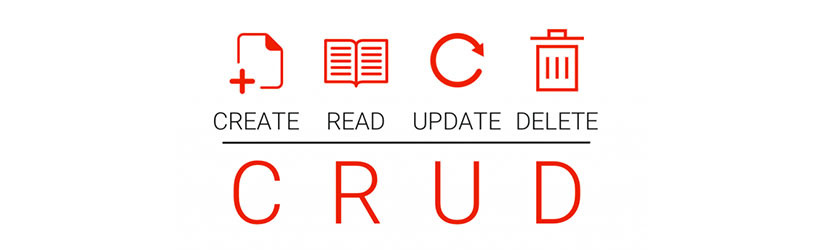
Introduction
The term CRUD is related to the management of digital data. It summarizes the operations or functions that a user needs to create and manage data.
The acronym CRUD stands for the four basic operations for interacting with database applications:
- Create : Create a data.
- Read : Read a data.
- Update : Update a data.
- Delete : Delete a data.
CRUD, SQL and HTTP
Each component of the CRUD acronym can be associated with a type of query in SQL as well as an HTTP1,2 method :
Operation | SQL | HTTP |
---|---|---|
Create | INSERT | POST / PUT |
Read | SELECT | GET |
Update | UDPATE | PATCH / PUT |
Delete | DELETE | DELETE |
As you can see, we can associate CRUD method with HTTP method. That's why CRUD design is extremly used in REST APIs.
CRUD and Laravel Resource
Laravel can handle the CRUD design by default. In Laravel (and in general) that's called a Resource. A Resource is represented by a Model controlled by the CRUD design of a Controller and Routes. Laravel artisan has a command to generate all the CRUD actions of a controller and all related routes :
php artisan make:controller PhotoController --resource
This command will generate the following code :
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class PhotoController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
We can translate the controller code to this array :
Method | URI | Action | Route Name | Description | Used in REST APIs |
---|---|---|---|---|---|
GET | /photos | index | photos.index | Display all photos | ![]() |
GET | /photos/create | create | photos.create | Show the form for creating a photo | ![]() |
POST | /photos | store | photos.store | Create a photo | ![]() |
GET | /photos/{photo} | show | photos.show | Display a photo | ![]() |
GET | /photos/{photo}/edit | edit | photos.edit | Show the form for editing a photo | ![]() |
PUT/PATCH | /photos/{photo} | update | photos.update | Edit a photo | ![]() |
DELETE | /photos/{photo} | destroy | photos.destroy | Delete a photo | ![]() |